投稿の一覧ページの投稿のタイトルを選択して、投稿の表示ページをを表示させます。
親記事
Dockerで環境構築して Laravel 9で CRUD 機能を作る
2022年03月15日
Laravel前記事
Laravel 9 投稿CRUD 機能を作成 〜 投稿を保存
2023年01月10日
Laravel環境
- macOS
- Laravel v9.0.2
- Docker 20.10.12
投稿を表示
PostController の show メソッドで 投稿を表示させます。
public function show(Post $post)
{
return view('posts.show', ['post' => $post]);
}
resources/views/posts/show.blade.php 表示ビューを作成します。
<x-app-layout>
<x-slot name="header">
<h2 class="font-semibold text-xl text-gray-800 leading-tight">
{{ __('Show') }}
</h2>
</x-slot>
<div class="py-12">
<div class="max-w-7xl mx-auto sm:px-6 lg:px-8">
<div class="bg-white shadow-sm sm:rounded-lg mb-10">
<div x-data="{toggle : false}" class="p-6 flex items-center justify-between">
<a href="{{ route('posts.edit', $post->id) }}">
<button class="rounded bg-green-400 text-white py-2 px-3">編集</button>
</a>
<button x-show="!toggle" @click="toggle = ! toggle" class="p-2 bg-blue-400 rounded text-white">削除</button>
<div x-show="toggle" @click.away="toggle = false" class="flex items-center mx-3 justify-between" x-transition:enter="transition transform ease-out duration-300" x-transition:enter-start="-translate-x-5 opacity-0" x-transition:enter-end="translate-x-0">
<form method="post" action="{{ route('posts.destroy', $post->id) }}">
@csrf
@method('DELETE')
<button type="submit" class="py-2 px-3 bg-red-400 rounded-lg text-white">決定</button>
</form>
<button @click="toggle = false" class="p-2 mx-3 rounded-sm shadow-sm bg-yellow-100">キャンセル</button>
<p class="text-red-300 ">本当にいいですか?</p>
</div>
</div>
</div>
<div class="flex flex-col p-6 bg-white shadow-lg rounded-lg ">
<div class="flex my-3 py-2 items-center justify-between border-b-2">
<h2 class="text-lg font-semibold text-gray-900 -mt-1">{{ $post->title }}</h2>
<small class="text-sm text-gray-700">{{ $post->updated_at }}</small>
</div>
<p class="text-gray-700">{{ $post->user->name }}</p>
<p class="my-3 text-gray-700 text-sm">
{{ $post->content }}
</p>
</div>
</div>
</div>
</x-app-layout>
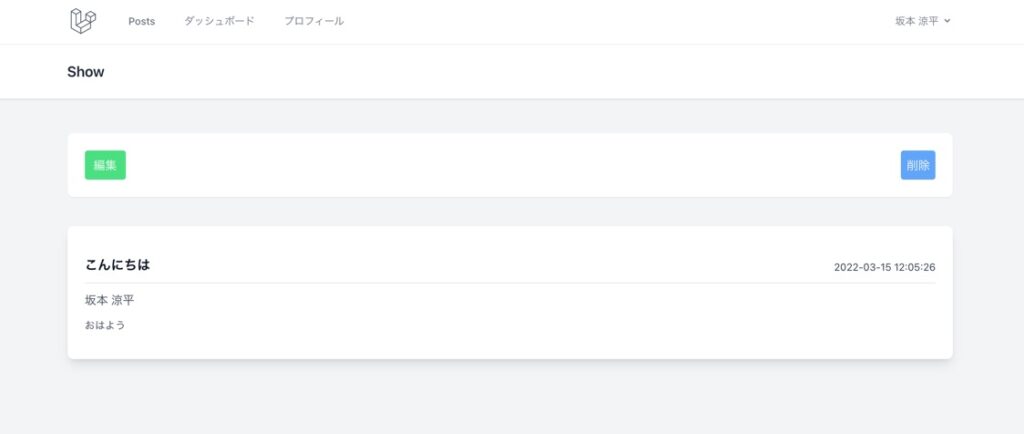
投稿が表示されました。
編集ボタンと削除ボタンを現在認証済みにのユーザーかつ、投稿をしたユーザーである場合にのみ表示させていきます。
ポリシー
ポリシーは、特定のモデルに関する認可処理を行うクラスです。Postモデルに関するので、PostPolicyを作成します。
php artisan make:policy PostPolicy
//sail
sail artisan make:policy PostPolicy
app/Policies ディレクトリにPostPolicy.php ファイルが作成されます。
ポリシーを登録
app/Providers/AuthServiceProvider.php ファイルでポリシーを登録します。
protected $policies = [
User::class => UserPolicy::class,
Post::class => PostPolicy::class,
];
PostPolicy
<?php
namespace App\Policies;
use App\Models\User;
use App\Models\Post;
use Illuminate\Auth\Access\HandlesAuthorization;
class PostPolicy
{
use HandlesAuthorization;
public function before(User $user, $ability)
{
$user_policy = new UserPolicy;
if ($user_policy->isAdmin($user)) {
return true;
}
}
public function edit(User $user, Post $post)
{
return $user->id == $post->user_id;
}
}
14-20: 管理者には全ての許可を与えます。管理者は、UserPolicy ですでに定義済みです。
22-25: 現在の認証済みユーザーが、投稿を行ったユーザーかを判断します。
Laravelポリシーで管理者を設定する方法
2023年01月10日
Laravelビューに適用する
<x-app-layout>
<x-slot name="header">
<h2 class="font-semibold text-xl text-gray-800 leading-tight">
{{ __('Show') }}
</h2>
</x-slot>
<div class="py-12">
<div class="max-w-7xl mx-auto sm:px-6 lg:px-8">
<div class="bg-white shadow-sm sm:rounded-lg mb-10">
<div x-data="{toggle : false}" class="p-6 flex items-center justify-between">
@auth
@can('edit', $post)
<a href="{{ route('posts.edit', $post->id) }}">
<button class="rounded bg-green-400 text-white py-2 px-3">編集</button>
</a>
<button x-show="!toggle" @click="toggle = ! toggle" class="p-2 bg-blue-400 rounded text-white">削除</button>
<div x-show="toggle" @click.away="toggle = false" class="flex items-center mx-3 justify-between" x-transition:enter="transition transform ease-out duration-300" x-transition:enter-start="-translate-x-5 opacity-0" x-transition:enter-end="translate-x-0">
<form method="post" action="{{ route('posts.destroy', $post->id) }}">
@csrf
@method('DELETE')
<button type="submit" class="py-2 px-3 bg-red-400 rounded-lg text-white">決定</button>
</form>
<button @click="toggle = false" class="p-2 mx-3 rounded-sm shadow-sm bg-yellow-100">キャンセル</button>
<p class="text-red-300 ">本当にいいですか?</p>
</div>
@endcan
@endauth
</div>
</div>
<div class="flex flex-col p-6 bg-white shadow-lg rounded-lg ">
<div class="flex my-3 py-2 items-center justify-between border-b-2">
<h2 class="text-lg font-semibold text-gray-900 -mt-1">{{ $post->title }}</h2>
<small class="text-sm text-gray-700">{{ $post->updated_at }}</small>
</div>
<p class="text-gray-700">{{ $post->user->name }}</p>
<p class="my-3 text-gray-700 text-sm">
{{ $post->content }}
</p>
</div>
</div>
</div>
</x-app-layout>
13, 31: @auth ~ @endauth 認証済みユーザーでない場合非表示にします。
14,32: Blade内でポリシーを使う場合は、@can ~ @endcan および@cannot ~ @endcannotを使用できます。 PostPlicyのeditメソッドを呼び出し、投稿したユーザーと一致しない場合に非表示にします。
次の記事
Laravel9 投稿 CRUD機能を作成 〜 編集と削除
2023年01月10日
Laravel