パスワードフォームで使われる目のマークを選択すると、伏せ字のパースワードが表示される機能を実装していきます。目のマークをクリックすると目が閉じているアイコンに変更して、フォームの型をpassword から text に変更すると、実装出来ます。
アイコンを用意する
fontawesome を使用してアイコンを用意します。
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/css/all.min.css" integrity="sha512-KfkfwYDsLkIlwQp6LFnl8zNdLGxu9YAA1QvwINks4PhcElQSvqcyVLLD9aMhXd13uQjoXtEKNosOWaZqXgel0g==" crossorigin="anonymous" referrerpolicy="no-referrer" />
コードを head タグに追加します。
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/css/all.min.css"
integrity="sha512-KfkfwYDsLkIlwQp6LFnl8zNdLGxu9YAA1QvwINks4PhcElQSvqcyVLLD9aMhXd13uQjoXtEKNosOWaZqXgel0g=="
crossorigin="anonymous" referrerpolicy="no-referrer" />
<title>Form</title>
</head>
fontawesome でアイコンを選択します。
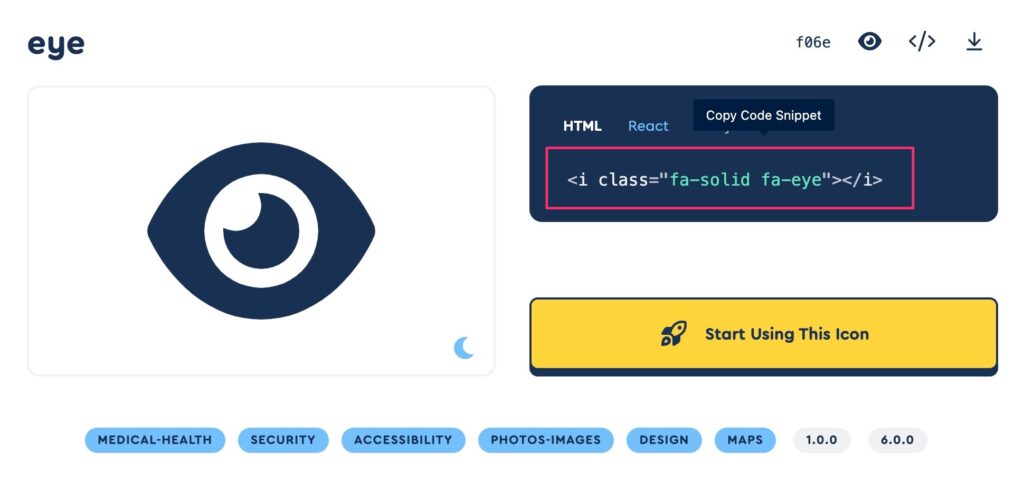
i 要素を使う方法でアイコンを使用します。
<i class="fa-solid fa-eye"></i>
目が開いているアイコン
<i class="fa-solid fa-eye-slash"></i>
目が閉じているアイコン
class を変更して、アイコンの見た目を変更していきます。
<form action="/" method="GET">
<div class="form-wrapper">
<input type="password">
<i id="eye" class="fa-solid fa-eye"></i>
</div>
</form>
4: アイコンのタグに id を追加します。
見た目を整える
.form-wrapper {
display: flex;
justify-content: center;
gap: 10px;
margin: 10px 0;
width: 250px;
}
#eye {
display: flex;
align-items: center;
width: 25px;
height: 25px;
cursor: pointer;
}
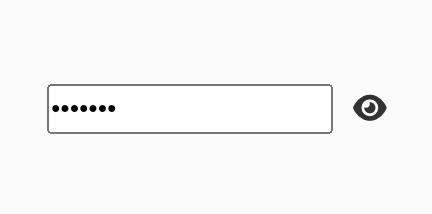
クリックイベント
let eye = document.getElementById("eye");
eye.addEventListener('click', function () {
if (this.previousElementSibling.getAttribute('type') == 'password') {
this.previousElementSibling.setAttribute('type', 'text');
this.classList.toggle('fa-eye');
this.classList.toggle('fa-eye-slash');
} else {
this.previousElementSibling.setAttribute('type', 'password');
this.classList.toggle('fa-eye');
this.classList.toggle('fa-eye-slash');
}
})
1: アイコンに追加した id を指定します。
2: クリックイベントを設定します。
3: クリックした要素の前の要素(フォーム)の type 属性が password の場合
4: 前の要素の type 属性を text に変更
5: クリックされたアイコンの class に fa-eye がある場合削除、ない場合追加する。
6: クリックされたアイコンの class に fa-eye-slash がある場合削除、ない場合追加する。
3-4: でフォームの型の変更、5-6: でアイコンの変更を行っています。
以上 HTML パスワードフォームの目のマークを実装する方法でした。
全体のコード
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.1/css/all.min.css"
integrity="sha512-KfkfwYDsLkIlwQp6LFnl8zNdLGxu9YAA1QvwINks4PhcElQSvqcyVLLD9aMhXd13uQjoXtEKNosOWaZqXgel0g=="
crossorigin="anonymous" referrerpolicy="no-referrer" />
<title>Form</title>
</head>
<body>
<form action="/" method="GET">
<div class="form-wrapper">
<input type="password">
<i id="eye" class="fa-solid fa-eye"></i>
</div>
</form>
<script>
</script>
<style>
.form-wrapper {
display: flex;
justify-content: center;
gap: 10px;
margin: 10px 0;
width: 250px;
}
#eye {
display: flex;
align-items: center;
width: 25px;
height: 25px;
cursor: pointer;
}
</style>
<script>
let eye = document.getElementById("eye");
eye.addEventListener('click', function () {
if (this.previousElementSibling.getAttribute('type') == 'password') {
this.previousElementSibling.setAttribute('type', 'text');
this.classList.toggle('fa-eye');
this.classList.toggle('fa-eye-slash');
} else {
this.previousElementSibling.setAttribute('type', 'password');
this.classList.toggle('fa-eye');
this.classList.toggle('fa-eye-slash');
}
})
</script>
</body>
</html>